Quick Start
Introduction
The quick start includes short examples to get you up and running with the Robot Raconteur communication framework, fast!
The quick start examples use the Reynard the Robot package, a simple cartoon robot designed to help learn Robot Raconteur. The examples include a simple service, and clients in Python, MATLAB, and LabView.
All quick start example files can be found in the examples/quickstart
directory.
Setup
Before running the examples, install Python 3.8 or greater. At the time of this writing, the current Python version
is Python 3.11. Python can be downloaded from the Python Download Page,
or installed using a package manager such as apt
.
Install the required packages using pip
python -m pip install --user robotraconteur pyserial opencv-contrib-python
Note
On Ubuntu, Debian, and other versions of Linux, replace python
with python3
in all examples. It may
also be necessary to install the python3-pip
package.
The robotraconteur
Python package is available for most platforms using the pip
installer, but for some
platforms it needs to be installed separately. See the
Robot Raconteur Install
page for more information on these platforms.
Reynard the Robot Quick Start
The Reynard the Robot quick start creates a simple service, and clients in Python, MATLAB, and LabView. The following sections describe the examples. Use a web browser pointed to http://localhost:29201 to view the Reynard the Robot user interface.
Reynard the Robot Quick Start Python Service
The example uses a “service definition” with a single object, ReynardQuickstart
, and two functions
say
and teleport
. The say
function takes the argument text
and displays it in the chat
window on the Reynard page. The teleport
function takes the arguments x
and y
and moves the
Reynard robot base to the new location.
service experimental.reynard_quickstart
object ReynardQuickstart
function void say(string text)
function void teleport(double x, double y)
end
More information about Service Definitions can be found in the Service Definition Framework Documentation page.
Now, a simple service written in Python can be created:
# reynard_quickstart_service.py
import RobotRaconteur as RR
RRN = RR.RobotRaconteurNode.s
import threading
import reynard_the_robot
# Define the service definition for the quickstart service
reynard_quickstart_interface = """
service experimental.reynard_quickstart
object ReynardQuickstart
function void say(string text)
function void teleport(double x, double y)
end
"""
# Implement the quickstart service
class ReynardQuickstartImpl(object):
def __init__(self):
self.reynard = reynard_the_robot.Reynard()
self.reynard.start()
self._lock = threading.Lock()
def say(self, text):
with self._lock:
self.reynard.say(text)
def teleport(self, x, y):
with self._lock:
self.reynard.teleport(x, y)
with RR.ServerNodeSetup("experimental.reynard_quickstart", 53222):
# Register the service type
RRN.RegisterServiceType(reynard_quickstart_interface)
reynard_inst = ReynardQuickstartImpl()
# Register the service
RRN.RegisterService("reynard", "experimental.reynard_quickstart.ReynardQuickstart", reynard_inst)
# Wait for program exit to quit
input("Press enter to quit")
Reynard Quick Start Clients
The Reynard Quick Start service can now be called by connecting a client. Because of the magic of Robot Raconteur, it is only necessary to connect to the service to utilize its members. In Python and MATLAB there is no boilerplate code, and in the other languages the boilerplate code is generated automatically.
# reynard_quickstart_client.py
from RobotRaconteur.Client import *
# RRN is imported from RobotRaconteur.Client
# Connect to the service.
obj = RRN.ConnectService('rr+tcp://localhost:53222/?service=reynard')
# Call the say function
obj.say("Hello from Reynard!")
# Call the teleport function
obj.teleport(100, 200)
In MATLAB, this client is even simpler.
% reynard_quickstart_client.m
% Connect to the service
o = RobotRaconteur.ConnectService('rr+tcp://localhost:53222/?service=reynard');
% Call the say function
o.say("Hello from MATLAB!");
% Call the teleport function
o.teleport(-150,200);
The Robot Raconteur Matlab add-on can be installed using “Get Add-Ons” and “Add-On Explorer” in MATLAB.
LabView can also be used to drive the robot:
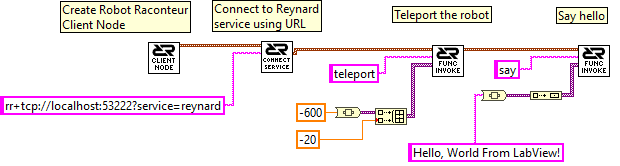
The Robot Raconteur LabView toolbox is a commercial product available from Wason Technology, LLC.
These example clients all use a URL to connect to the robot. “Discovery” and “Subscriptions” can also be used to connect automatically to devices, even if the URL is not known.
# reynard_quickstart_client_sub.py
from RobotRaconteur.Client import *
# RRN is imported from RobotRaconteur.Client
# Create a subscription and connect to the default client
sub = RRN.SubscribeService('rr+tcp://localhost:53222/?service=reynard')
obj = sub.GetDefaultClientWait(5)
# Call the say function
obj.say("Hello from Reynard!")
# Call the teleport function
obj.teleport(100, 200)
Subscriptions can use “Filters” to select which service to connect. See the documentation for SubscribeServiceByType for more information on filters.
Service Browser
The Robot Raconteur Service Browser is a utility to browse services available on the network and find connection information.
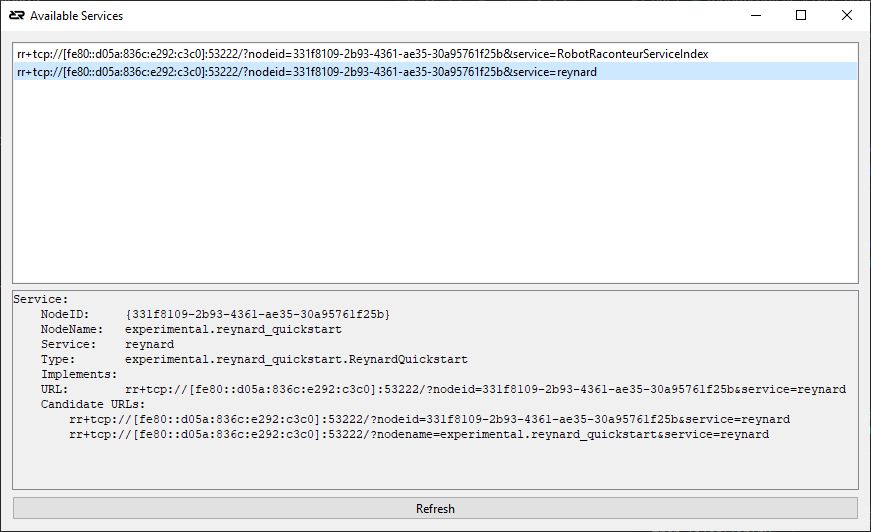
The URL can be cut and paste from the information display. Note that this URL uses an IPv6 link-local address
fe80::d05a:836c:e292:c3c0
instead of the more common IPv4 address. IPv6 addresses are favored by Robot Raconteur
since the they do not require as much configuration as IPv4 when working on a local network and are more reliable.